Build pages in Gatsby from Rest API
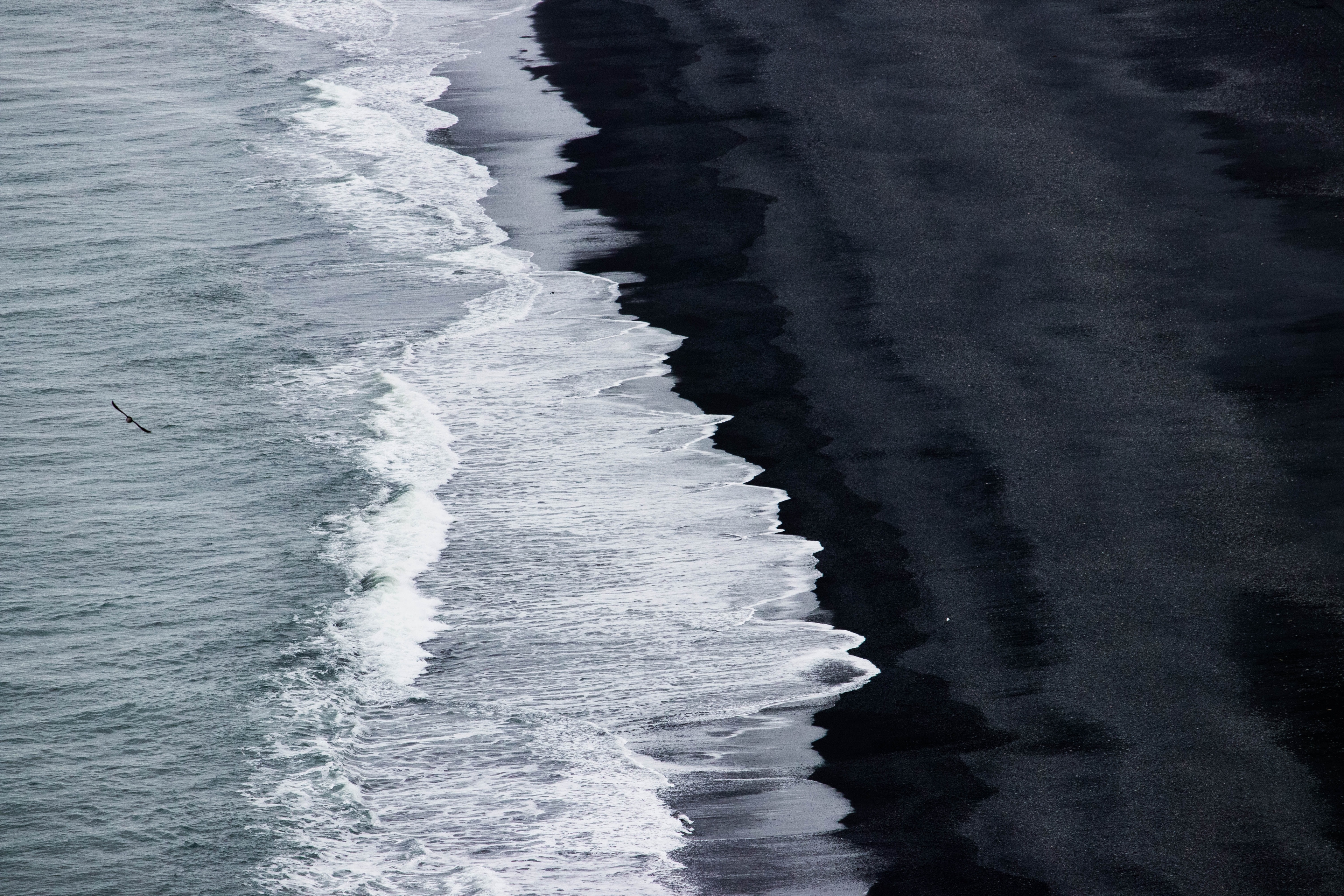
Photo by Tamara Bitter on Unsplash
Creating pages in Gatsby is easy. But when we want the pages to be also part of the GraphQL layer so that we can query them in index pages, we need to hook into sourceNode()
in the gatsby-node.js
.
js1// gatsby-node.js23const fetch = require(‘node-fetch’;)45// part one6const createPages = async ({ actions }) => {7 const { createPage } = actions;89 const allPosts = await (await fetch(‘http://some-api.com/all’)).json();10 for (const post of allPosts) {11 const blocks = await (await fetch(‘http://some-api.com/post/’ + post.id)).json();12 createPage({13 path: `writings/${post.Slug}`,14 component: path.resolve(`./src/templates/post.js`),15 context: {16 slug: `writings/${post.Slug}`,17 blocks,18 },19 });20 }21};2223// part two24const sourceNodes = async ({ actions }) => {25 const { createNode } = actions;2627 const allPosts = await (await fetch(‘http://some-api.com/all’)).json();28 for (const post of allPosts) {29 // create node for graphql30 const node = {31 id: `${post.Slug}`,32 parent: `__SOURCE__`,33 internal: {34 // lets you query nodes using allAPIPost and APIPost35 type: `APIPost`,36 },37 children: [],3839 // Other fields that you want to query with graphQl40 slug: post.Slug,41 title: post.Name,42 date: post.Date,43 draft: post.Draft,44 };45 const contentDigest = crypto.createHash(`md5`).update(JSON.stringify(node)).digest(`hex`);46 node.internal.contentDigest = contentDigest;47 createNode(node);48 }49};5051// module.exports = { createPages, sourceNodes };52
Let me explain this. There are 2 parts to it.
- One - creating the pages after fetching from the rest API using
createPage()
- Two - creating the node in the GraphQL layer to be able to query in other pages (for example in
index.js
where you list all the pages)
After adding that piece of code to gatsby-node.js
and running gatsby develop
you will have pages created at the path
you’ve given to createPage()
.
To list them in any other page, we can use a query that looks like this
graphql1query {2 allPost(sort: { order: DESC, fields: date }) {3 nodes {4 slug5 title6 date7 }8 }9}10
You can experiment with the query in GraphiQL dashboard that runs when you gatsby develop
.
Hope this helps.
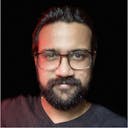
By Aravind Balla, a Javascript Developer building things to solve problems faced by him & his friends. You should hit him up on Twitter!