Gatsby without GraphQL - only JSON
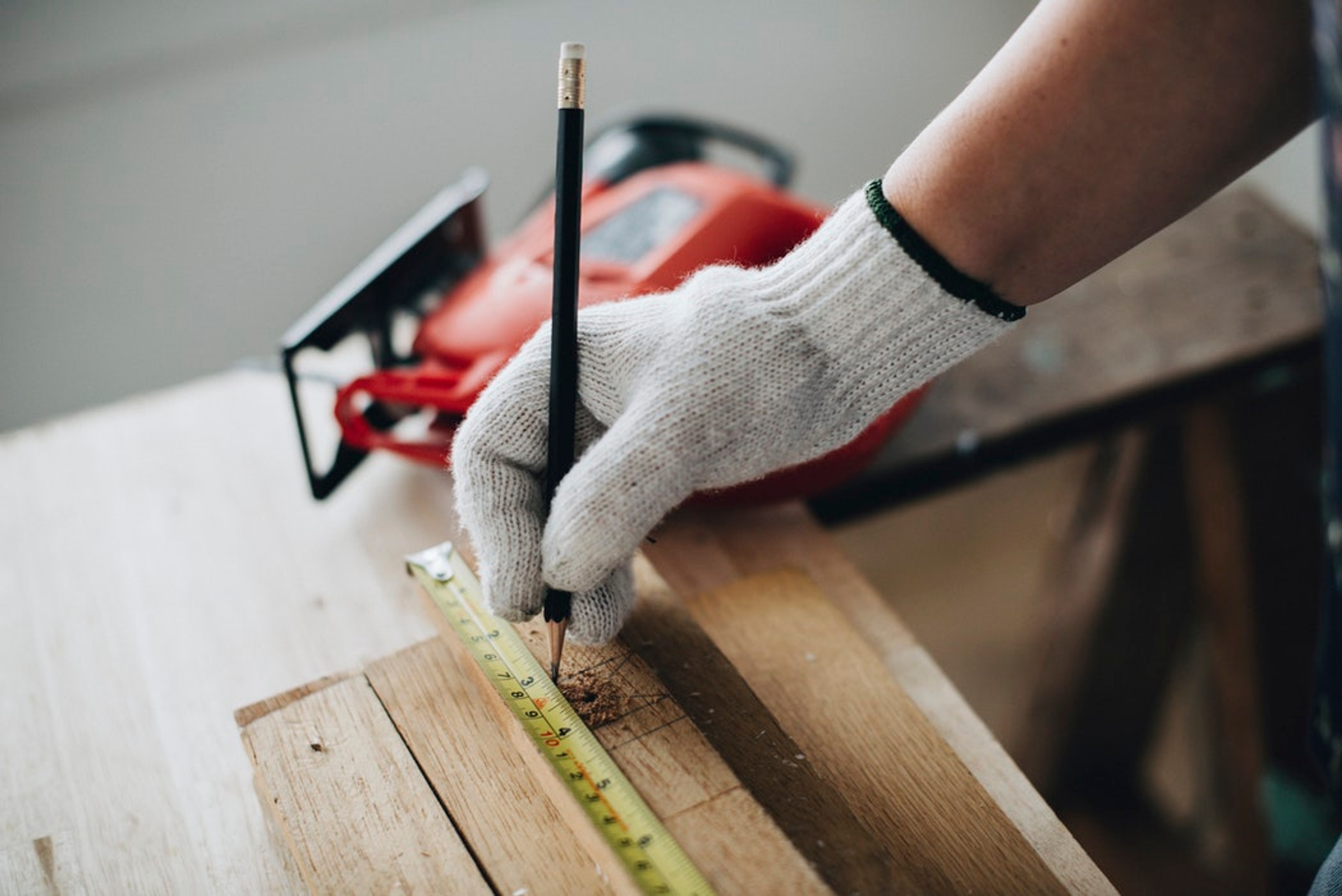
Number one misconception about Gatsby is that you need to know GraphQL to build something with Gatsby, which is not true. Yes, you don't need GraphQL. A Gatsby site can be built from an API or just with some data from a JSON file.
You don't need GraphQL.
If you don't know what Gatsby is, it is a static site generator built in React. You can use React to build the components of the website and give them to Gatsby, which will then generate a blazing fast website for you.
If you are interested in building it from an API, here is a well demonstrated example.
If most of you site is static and only certain part of it changes, why would you need GraphQL to fetch things.
Let us build a site from just a JSON file which contains some data.
Let's Start
Step One is to clone the hello-world-starter
and make sure everything works.
bash1npx gatsby new hello-world https://github.com/gatsbyjs/gatsby-starter-hello-world2cd hello-world3yarn develop4
Now, http://localhost:8000
should say, "hello world!".
Adding the data
Add a JSON file at content/data.json
which has information on the pages. The path is just a personal preference ;)
javascript1// content/data.json2{3 "pages": [4 {5 "title": "About",6 "slug": "about",7 "paragraphs": [8 {9 "heading": "Work",10 "content": "Works as a Javascript Enginneer. Has experience with Gatsby, React, Javascript, Node, Mongo and a little Python as well"11 }12 ]13 },14 {15 "title": "Talks",16 "slug": "talks",17 "paragraphs": [18 {19 "heading": "Building A Progressive Web App",20 "content": "PWA (Progre..."21 },22 ...23 ]24 }25 ]26}27
Template to render the Page
Next, we create a template which will get the data from the JSON and render it to the webpage.
javascript1// src/templates/page.js2import React from 'react';34const PageTemplate = (props) => {5 const { title = null, paragraphs = null } = props.pageContext;67 return (8 <React.Fragment>9 {title && <h1>{title}</h1>}10 {paragraphs &&11 paragraphs.map((para) => (12 <div>13 <h2>{para.heading}</h2>14 <p>{para.content}</p>15 </div>16 ))}17 </React.Fragment>18 );19};2021export default PageTemplate;22
A very naive and basic React component which gets title
and paragraphs
in the pageContext
and it renders them. We will get to how the data is passed to pageContext
in a while.
Create the Pages now!
How do we tell Gatsby what pages it should create? By creating gatsby-node.js
!
javascript1// gatsby-node.js2const path = require(`path`);3const fs = require('fs');45exports.createPages = ({ actions }) => {6 const { createPage } = actions;78 const pageData = JSON.parse(fs.readFileSync('./content/data.json', { encoding: 'utf-8' }));9 const blogPostTemplate = path.resolve(`src/templates/page.js`);1011 pageData.pages.forEach((page) => {12 createPage({13 path: page.slug,14 component: blogPostTemplate,15 context: {16 ...page,17 },18 });19 });20};21
Here, we use createPages()
API, where we loop through all the pages from the JSON data, and call createPage()
action to create the actual page. Here we pass the template(which is component) and the URL(path) where the page has to be generated. The actual data is passed as context
. This is the context that we use in our Page template to populate the data.
Run
Boom! It actually works of you have used yarn develop
. It hot reloads every change you make. Now you can navigate to http://localhost:8000/talks
and /about
pages and see how they look (and feel good about yourself).
You realise the potential of Gatsby, right? Possibilities are limitless. In this case, you just have to add styles and you have a portfolio site.
Thanks for reading through.
Keep on hacking!
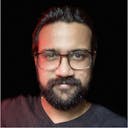
By Aravind Balla, a Javascript Developer building things to solve problems faced by him & his friends. You should hit him up on Twitter!